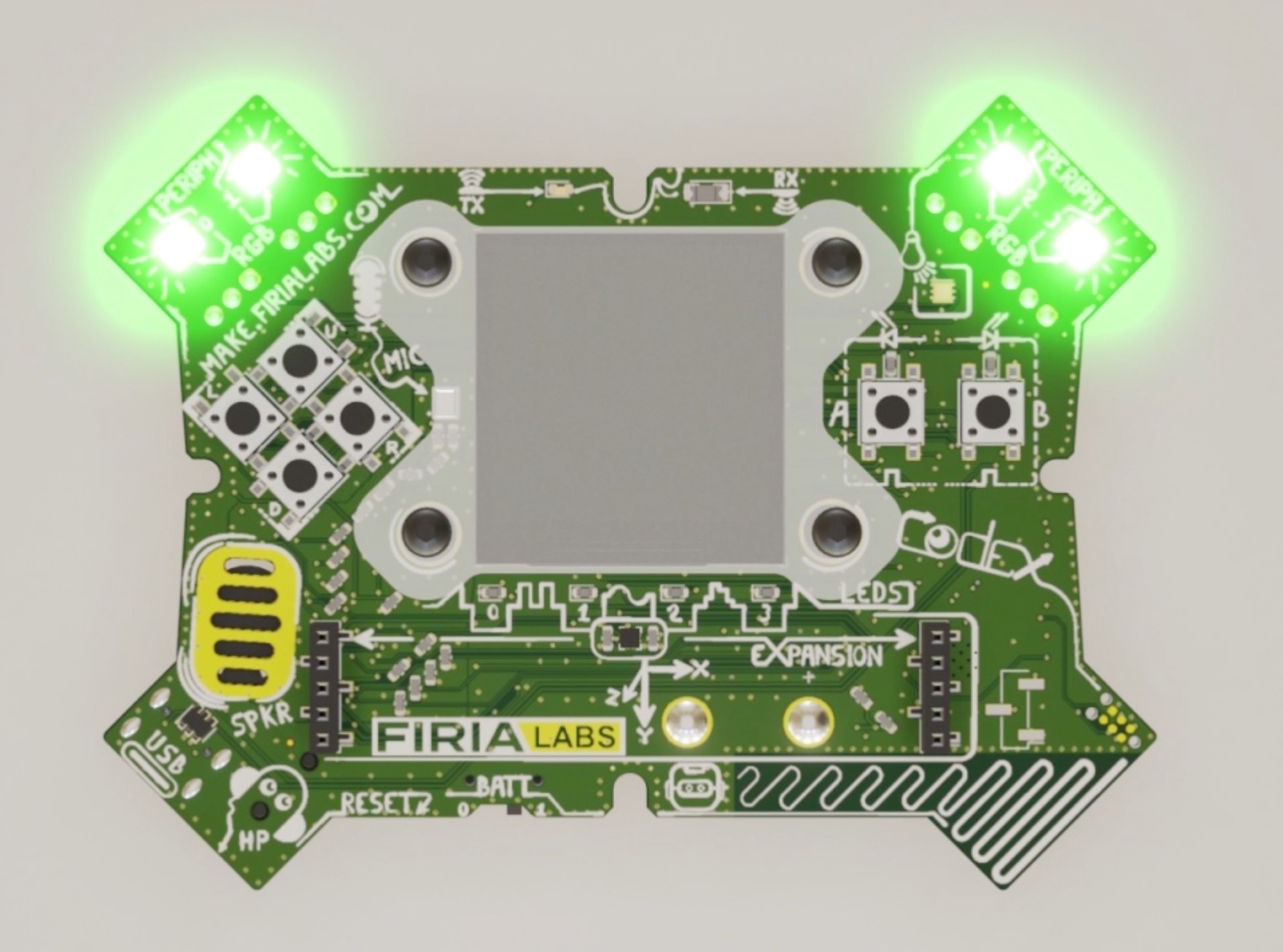
NeoPixels¶
Across the top two points of the X shape on your CodeX are super-bright color LEDs. These are of a type commonly found in LED strips you may have seen lighting up the walls or ceiling of a room. Among makers they’re often referred to as “NeoPixels” which is what we’ll call them here (credit to Adafruit for that name!) but the exact parts on the CodeX are WS2813-mini LEDs.
Warning
These lights can be VERY BRIGHT! Please exercise caution when you use higher brightness levels, and don’t stare directly into the lights.
1. Lighting up a Pixel¶
Each WS2813 pixel contains 3 LEDs: RED, GREEN, and BLUE. These can be controlled with variable intensity, allowing you to create millions of possible colors!
You can define a color using a (red, green, blue) tuple
, with each value ranging from 0-255.
For example, color = (50, 0, 0)
would be pure red at about 1/5 the full brightness range.
Take a look at the codex.NEOPixels
documentation for details on how to program these brilliant components!
The following code lights up all 4 codex.NEOPixels
using built-in color indexes conveniently defined in module codex.colors
.
from codex import *
pixels.set(0, RED)
pixels.set(1, WHITE)
pixels.set(2, BLUE)
pixels.set(3, GREEN)
2. Scintillating Colors!¶
The above barely scratches the surface of these dazzling lights. Try the following program, which uses
Python’s random
module to cycle through random colors to amaze and delight you.
from codex import *
from time import sleep
from random import randrange
def get_random_color():
"""Select a random RGB value"""
red = randrange(256)
green = randrange(256)
blue = randrange(256)
return (red, green, blue)
while True:
pixels.set(0, get_random_color())
pixels.set(1, get_random_color())
pixels.set(2, get_random_color())
pixels.set(3, get_random_color())
sleep(0.1)